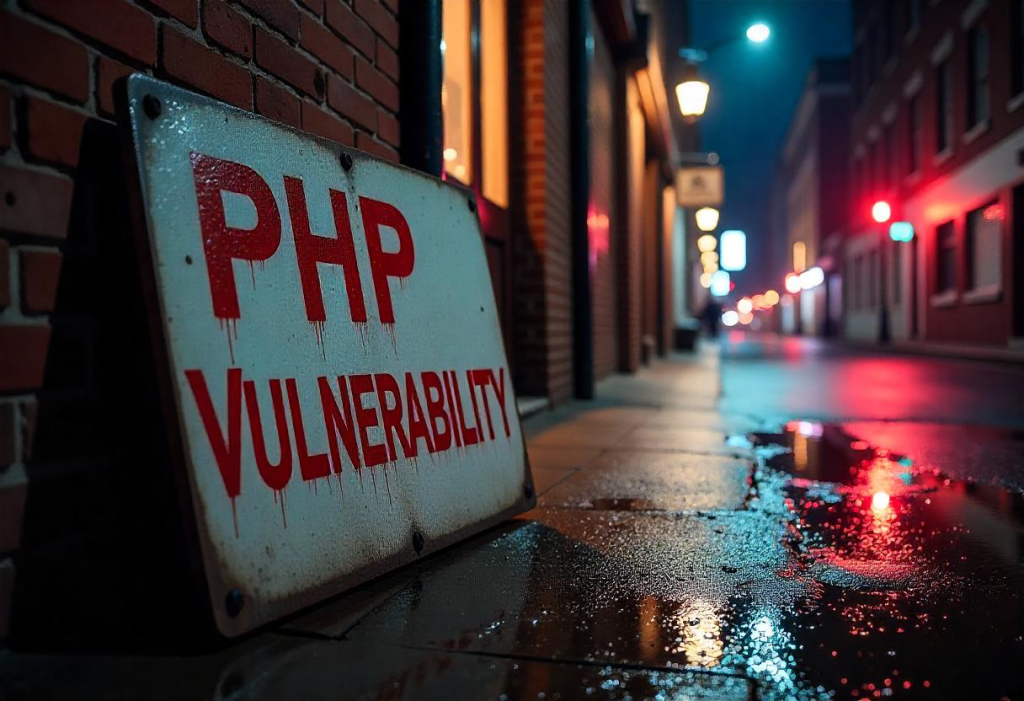
PHP remains one of the most widely used server-side scripting languages, powering millions of websites, including major platforms like WordPress, Facebook (historically), and Wikipedia. However, its popularity also makes it a prime target for cyberattacks. Poorly written PHP code can lead to serious security vulnerabilities, compromising sensitive data and system integrity.
In this article, I will show you some of the most common PHP security vulnerabilities and also provide practical solutions to mitigate them.
See How to Set Up a React Native Android Environment on MacBooks
1. SQL Injection (SQLi)
What is it?
SQL Injection occurs when an attacker inserts malicious SQL queries into input fields (like login forms or search boxes), allowing them to manipulate or extract database information.
Example of Vulnerable Code:
$username = $_POST['username'];
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE username = '$username' AND password = '$password'";
$result = mysqli_query($conn, $query);
If an attacker enters ' OR '1'='1
as the username, they could bypass authentication.
How to Fix It:
- Use Prepared Statements (PDO or MySQLi):
$stmt = $conn->prepare("SELECT * FROM users WHERE username = ? AND password = ?");
$stmt->bind_param("ss", $username, $password);
$stmt->execute();
- Escape Inputs (if not using PDO):
$username = mysqli_real_escape_string($conn, $_POST['username']);
- Use an ORM (e.g., Eloquent, Doctrine).
2. Cross-Site Scripting (XSS)
What is it?
XSS attacks occur when malicious JavaScript is injected into a webpage, often through user inputs, and executed in a victim’s browser.
Example of Vulnerable Code:
echo "<div>" . $_GET['comment'] . "</div>";
An attacker could submit:
<script>alert('XSS Attack!');</script>
How to Fix It:
- Use
htmlspecialchars()
to escape output:
echo "<div>" . htmlspecialchars($_GET['comment'], ENT_QUOTES, 'UTF-8') . "</div>";
- Implement Content Security Policy (CSP) headers.
- Use templating engines (Twig, Blade) that auto-escape by default.
3. Cross-Site Request Forgery (CSRF)
What is it?
CSRF tricks users into executing unwanted actions on a web application where they are authenticated (e.g., changing passwords or making payments).
Example of Vulnerable Code:
<form action="/change_password" method="POST">
<input type="password" name="new_password">
<button type="submit">Update Password</button>
</form>
An attacker could craft a malicious link or form submission.
How to Fix It:
- Use CSRF Tokens:
session_start();
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
if (!isset($_POST['csrf_token']) || $_POST['csrf_token'] !== $_SESSION['csrf_token']) {
die("CSRF validation failed.");
}
}
$_SESSION['csrf_token'] = bin2hex(random_bytes(32));
<input type="hidden" name="csrf_token" value="<?php echo $_SESSION['csrf_token']; ?>">
- Use frameworks (Laravel, Symfony) with built-in CSRF protection.
4. File Inclusion Vulnerabilities (LFI/RFI)
What is it?
- Local File Inclusion (LFI): Allows attackers to include local files (e.g.,
/etc/passwd
). - Remote File Inclusion (RFI): Allows including remote files (e.g., malicious scripts).
Example of Vulnerable Code:
$page = $_GET['page'];
include($page . '.php');
An attacker could exploit this with:
http://example.com/?page=../../etc/passwd
How to Fix It:
- Whitelist allowed files:
$allowed_pages = ['home', 'about', 'contact'];
if (in_array($_GET['page'], $allowed_pages)) {
include($_GET['page'] . '.php');
} else {
die("Invalid page request.");
}
- Disable
allow_url_include
inphp.ini
.
5. Session Hijacking & Fixation
What is it?
Attackers steal or manipulate session IDs to impersonate users.
Example of Vulnerable Code:
- Weak session ID generation.
- Sessions stored in unsafe locations.
How to Fix It:
- Use
session_regenerate_id()
on login:
session_start();
session_regenerate_id(true);
- Set secure session cookie attributes:
session_set_cookie_params([
'lifetime' => 3600,
'path' => '/',
'secure' => true, // HTTPS only
'httponly' => true, // Prevent JavaScript access
'samesite' => 'Strict' // Prevent CSRF
]);
6. Insecure File Uploads
What is it?
Allowing arbitrary file uploads can lead to remote code execution (e.g., uploading a .php
file).
How to Fix It:
- Validate file types:
$allowed_types = ['image/jpeg', 'image/png'];
if (!in_array($_FILES['file']['type'], $allowed_types)) {
die("Invalid file type.");
}
- Store files outside the web root.
- Rename uploaded files:
$new_name = bin2hex(random_bytes(16)) . '.jpg';
move_uploaded_file($_FILES['file']['tmp_name'], 'uploads/' . $new_name);
7. Using Outdated PHP Versions
Risk:
Older PHP versions (e.g., PHP 5.x) lack security patches, making them vulnerable to exploits.
Solution:
- Always use the latest stable PHP version (PHP 8.2+ recommended).
- Regularly update dependencies (Composer packages).
Best Practices for PHP Security
- Use HTTPS (via Let’s Encrypt or other SSL providers).
- Enable PHP Security Headers (e.g.,
X-Frame-Options
,X-Content-Type-Options
). - Disable Dangerous Functions in
php.ini
(e.g.,exec
,system
,shell_exec
). - Follow the Principle of Least Privilege (limit database/user permissions).
- Use Security Tools like:
- PHPStan (static analysis)
- RIPS (vulnerability scanner)
- OWASP ZAP (penetration testing)
Conclusion
PHP security is critical to protecting web applications from attacks. By following secure coding practices, validating inputs, escaping outputs, and keeping software updated, developers can significantly reduce risks.
Always test your code with security tools and stay informed about new vulnerabilities. A proactive approach to security will save you from costly breaches in the future.
- Common PHP Security Vulnerabilities and How to Fix Them - May 8, 2025
- How to Set Up a React Native Android Environment on MacBooks - April 26, 2025
Discover more from TruthPost
Subscribe to get the latest posts sent to your email.